SearchBar
Usage
Default SearchBar
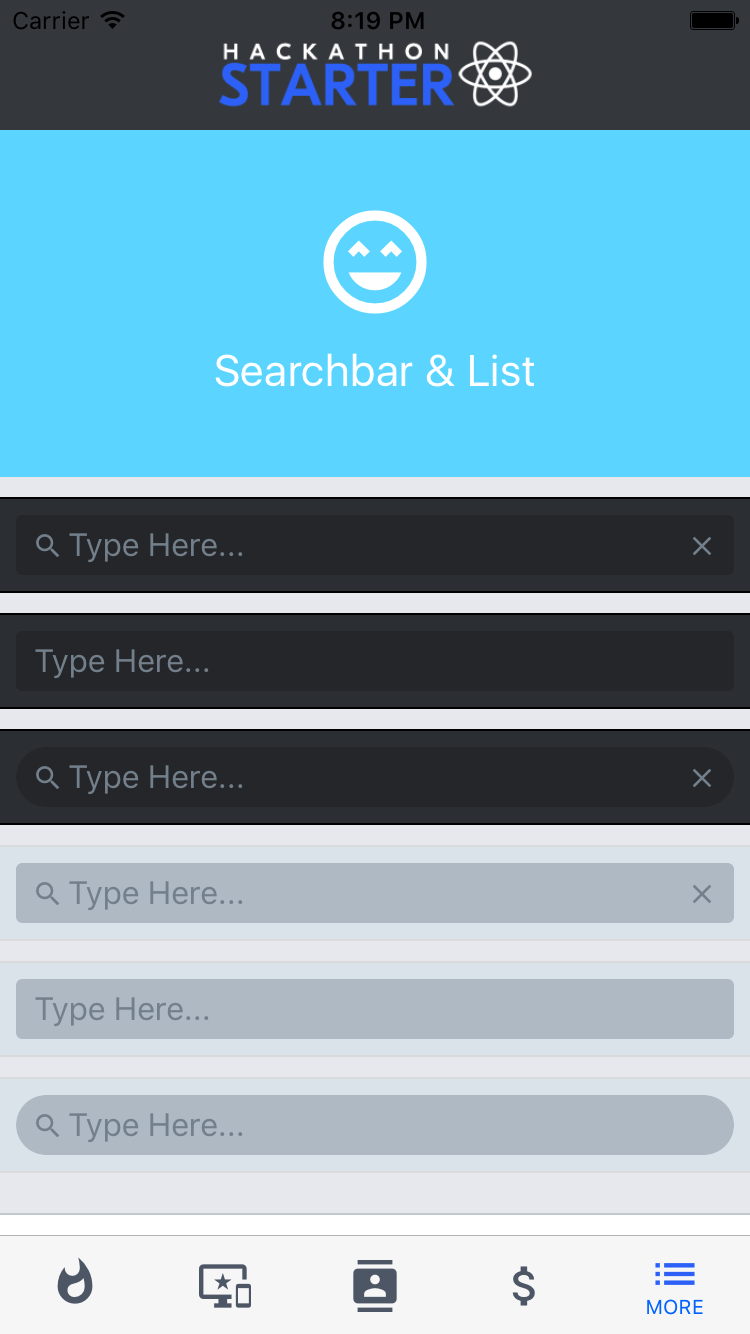
Platform specific SearchBar
iOS

Android

Interaction methods
method | description |
---|---|
focus | call focus on the textinput (example) |
blur | call blur on the textinput (example) |
clear | call clear on the textinput (example) |
cancel | (Android and iOS SearchBars only) call cancel on the SearchBar (left arrow on Android, Cancel button on iOS). This will basically blur the input and hide the keyboard (example) |
Calling methods on SearchBar
Store a reference to the SearchBar in your component by using the ref prop provided by React (see docs):
<SearchBar
ref={search => this.search = search}
...
/>
You can then access SearchBar methods like so:
this.search.focus();
this.search.blur();
this.search.clear();
this.search.cancel(); // Only available if `platform` props is "ios" | "android"
Props
note
Includes all Input, SearchBarBase props.
Name | Type | Default | Description |
---|---|---|---|
cancelButtonProps | Text Style | ||
cancelButtonTitle | string | ||
cancelIcon | IconNode | ||
clearIcon | IconNode or ({ name: string; } & IconNode) | ||
containerStyle | View Style | ||
inputContainerStyle | View Style | ||
inputStyle | Text Style | ||
leftIconContainerStyle | View Style | ||
lightTheme | boolean | ||
loadingProps | ActivityIndicatorProps | ||
onBlur | (() => void) or (((e: NativeSyntheticEvent<TextInputFocusEventData>) => void) & (() => void)) or ((() => any) & (() => void) & ((e: NativeSyntheticEvent<TextInputFocusEventData>) => void)) or ((() => any) & ... 1 more ... & (() => void)) | Callback that is called when the text input is blurred | |
onCancel | (() => void) or ((() => any) & (() => void)) | ||
onChangeText | ((text: string) => void) or (((text: string) => void) & ((text: string) => void)) or ((() => any) & ((text: string) => void) & ((text: string) => void)) or ((() => any) & ((text: string) => void) & ((text: string) => void)) | Callback that is called when the text input's text changes.Changed text is passed as an argument to the callback handler. | |
onClear | (() => void) or ((() => any) & (() => void)) or ((() => any) & (() => void)) | ||
onFocus | (() => void) or (((e: NativeSyntheticEvent<TextInputFocusEventData>) => void) & (() => void)) or ((() => any) & (() => void) & ((e: NativeSyntheticEvent<TextInputFocusEventData>) => void)) or ((() => any) & ... 1 more ... & (() => void)) | Callback that is called when the text input is focused | |
platform | default or ios or android | 'default' as const | |
rightIconContainerStyle | View Style | ||
round | boolean | ||
searchIcon | IconNode or ({ name: string; } & IconNode) | ||
showCancel | boolean | ||
showLoading | boolean |