ListItem
ListItems are used to display rows of information, such as a contact list, playlist, or menu. They are very customizable and can contain switches, avatars, badges, icons, and more.
Usage
Default
Loading...
Icon
Loading...
Avatar
Loading...
LinearGradient
Loading...
Accordion
Use as a collapsible list item refer props for ListItem.Accordion
Loading...
Swipeable
Try swiping list item, refer props for ListItem.Swipeable
Loading...
Checkbox
Refer props for ListItem.CheckBox
Loading...
Using Map Function - Implemented with avatar
import { ListItem, Avatar } from '@rneui/themed'
const list = [
{
name: 'Amy Farha',
avatar_url: 'https://s3.amazonaws.com/uifaces/faces/twitter/ladylexy/128.jpg',
subtitle: 'Vice President'
},
{
name: 'Chris Jackson',
avatar_url: 'https://s3.amazonaws.com/uifaces/faces/twitter/adhamdannaway/128.jpg',
subtitle: 'Vice Chairman'
},
... // more items
]
<View>
{
list.map((l, i) => (
<ListItem key={i} bottomDivider>
<Avatar source={{uri: l.avatar_url}} />
<ListItem.Content>
<ListItem.Title>{l.name}</ListItem.Title>
<ListItem.Subtitle>{l.subtitle}</ListItem.Subtitle>
</ListItem.Content>
</ListItem>
))
}
</View>
Using Map Function - Implemented with link and icon
import { ListItem, Icon } from '@rneui/themed'
const list = [
{
title: 'Appointments',
icon: 'av-timer'
},
{
title: 'Trips',
icon: 'flight-takeoff'
},
... // more items
]
<View>
{
list.map((item, i) => (
<ListItem key={i} bottomDivider>
<Icon name={item.icon} />
<ListItem.Content>
<ListItem.Title>{item.title}</ListItem.Title>
</ListItem.Content>
<ListItem.Chevron />
</ListItem>
))
}
</View>
Using RN FlatList - Implemented with link and avatar
import { ListItem, Avatar } from '@rneui/themed'
const list = [
{
name: 'Amy Farha',
avatar_url: 'https://s3.amazonaws.com/uifaces/faces/twitter/ladylexy/128.jpg',
subtitle: 'Vice President'
},
{
name: 'Chris Jackson',
avatar_url: 'https://s3.amazonaws.com/uifaces/faces/twitter/adhamdannaway/128.jpg',
subtitle: 'Vice Chairman'
},
... // more items
]
keyExtractor = (item, index) => index.toString()
renderItem = ({ item }) => (
<ListItem bottomDivider>
<Avatar source={{uri: item.avatar_url}} />
<ListItem.Content>
<ListItem.Title>{item.name}</ListItem.Title>
<ListItem.Subtitle>{item.subtitle}</ListItem.Subtitle>
</ListItem.Content>
<ListItem.Chevron />
</ListItem>
)
render () {
return (
<FlatList
keyExtractor={this.keyExtractor}
data={list}
renderItem={this.renderItem}
/>
)
}
Using RN FlatList - Implemented with custom avatar component.
import { ListItem, Avatar } from '@rneui/themed'
const list = [
{
name: 'Amy Farha',
subtitle: 'Vice President'
},
{
name: 'Chris Jackson',
avatar_url: 'https://s3.amazonaws.com/uifaces/faces/twitter/adhamdannaway/128.jpg',
subtitle: 'Vice Chairman'
},
... // more items
]
keyExtractor = (item, index) => index.toString()
renderItem = ({ item }) => (
<ListItem bottomDivider >
<Avatar title={item.name[0]} source={item.avatar_url && { uri: item.avatar_url }}/>
<ListItem.Content>
<ListItem.Title>{item.name}</ListItem.Title>
<ListItem.Subtitle>{item.subtitle}</ListItem.Subtitle>
</ListItem.Content>
<ListItem.Chevron />
</ListItem>
)
render () {
return (
<FlatList
keyExtractor={this.keyExtractor}
data={list}
renderItem={this.renderItem}
/>
)
}
ListItem implemented with custom View for Subtitle
import { ListItem, Avatar } from '@rneui/themed'
render () {
return (
<ListItem>
<ListItem.Content>
<ListItem.Title>Limited supply! Its like digital gold!</ListItem.Title>
<View style={styles.subtitleView}>
<Image source={require('../images/rating.png')} style={styles.ratingImage}/>
<Text style={styles.ratingText}>5 months ago</Text>
</View>
</ListItem.Content>
</ListItem>
)
}
styles = StyleSheet.create({
subtitleView: {
flexDirection: 'row',
paddingLeft: 10,
paddingTop: 5
},
ratingImage: {
height: 19.21,
width: 100
},
ratingText: {
paddingLeft: 10,
color: 'grey'
}
})
Badges
Example badge usage
<ListItem>
<Badge
value={3}
textStyle={{ color: 'orange' }}
containerStyle={{ marginTop: -20 }}
/>
</ListItem>
Linear gradient + Scale feedback
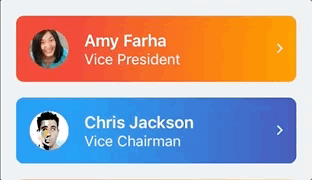
import { ListItem, Avatar } from '@rneui/themed';
import TouchableScale from 'react-native-touchable-scale'; // https://github.com/kohver/react-native-touchable-scale
import LinearGradient from 'react-native-linear-gradient'; // Only if no expo
<ListItem
Component={TouchableScale}
friction={90} //
tension={100} // These props are passed to the parent component (here TouchableScale)
activeScale={0.95} //
linearGradientProps={{
colors: ['#FF9800', '#F44336'],
start: { x: 1, y: 0 },
end: { x: 0.2, y: 0 },
}}
ViewComponent={LinearGradient} // Only if no expo
>
<Avatar rounded source={{ uri: avatar_url }} />
<ListItem.Content>
<ListItem.Title style={{ color: 'white', fontWeight: 'bold' }}>
Chris Jackson
</ListItem.Title>
<ListItem.Subtitle style={{ color: 'white' }}>
Vice Chairman
</ListItem.Subtitle>
</ListItem.Content>
<ListItem.Chevron color="white" />
</ListItem>;
Props
note
Includes all View props.
Name | Type | Default | Description |
---|---|---|---|
Component | React Component | Replace element with custom element. | |
ViewComponent | React Component | Container for linear gradient. | |
bottomDivider | boolean | Add divider at the bottom of the list item. | |
children | any | Add enclosed children. | |
containerStyle | View Style | Additional main container styling. | |
disabledStyle | View Style | Specific styling to be used when list item is disabled. | |
linearGradientProps | any | Props for linear gradient component. | |
pad | number | Adds spacing between the leftComponent, the title component & right component. | |
topDivider | boolean | Add divider at the top of the list item. |