SearchBar
SearchBars are used to search or filter items. Use a SearchBar when the number of items directly impacts a user's ability to find one of them.
Usage
Default SearchBar
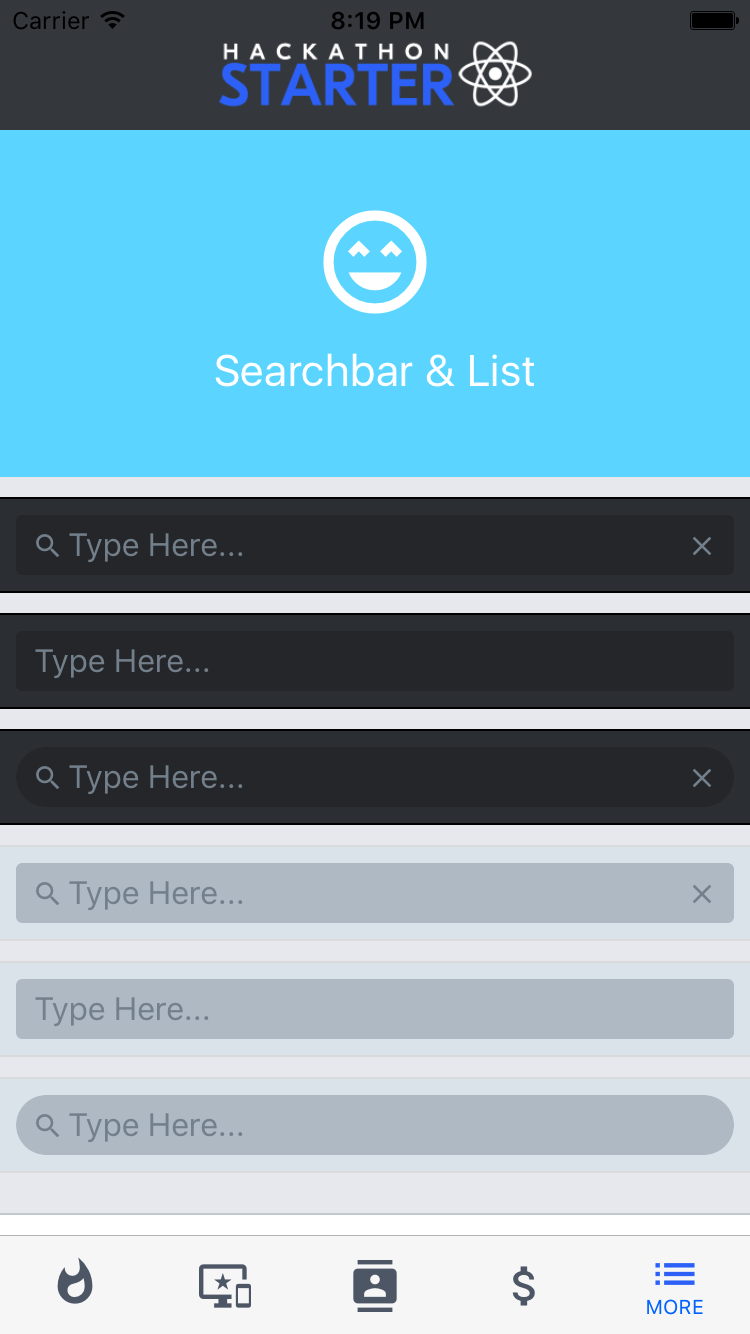
Platform specific SearchBar
iOS

Android

Interaction methods
method | description |
---|---|
focus | call focus on the textinput (example) |
blur | call blur on the textinput (example) |
clear | call clear on the textinput (example) |
cancel | (Android and iOS SearchBars only) call cancel on the SearchBar (left arrow on Android, Cancel button on iOS). This will basically blur the input and hide the keyboard (example) |
Calling methods on SearchBar
Store a reference to the SearchBar in your component by using the ref prop provided by React (see docs):
<SearchBar
ref={search => this.search = search}
...
/>
You can then access SearchBar methods like so:
this.search.focus();
this.search.blur();
this.search.clear();
this.search.cancel(); // Only available if `platform` props is "ios" | "android"
Props
This component inherits all React Native Elements Input props, which means all native TextInput props that come with a standard React Native TextInput element, along with the following:
cancelButtonProps
cancelButtonTitle
cancelIcon
(platform="android"
only)clearIcon
containerStyle
inputContainerStyle
inputStyle
leftIconContainerStyle
lightTheme
(platform="default"
only)loadingProps
onCancel
onChangeText
onClear
placeholder
placeholderTextColor
platform
rightIconContainerStyle
round
(platform="default"
only)searchIcon
showCancel
(platform="ios"
only)showLoading
underlineColorAndroid
Reference
cancelButtonProps
(iOS only) props passed to cancel Button
Also receives all TouchableOpacity props
buttonStyle
cancel Button styling
Type | Default |
---|---|
object (style) | none |
buttonTextStyle
cancel Button Text styling
Type | Default |
---|---|
object (style) | none |
color
cancel Button text color
Type | Default |
---|---|
string (color) | #007aff |
disabled
Prop to indicate cancel Button is disabled
Type | Default |
---|---|
boolean | false |
buttonDisabledStyle
Disabled cancel Button styling
Type | Default |
---|---|
object (style) | none |
buttonDisabledTextStyle
Styles for the text when cancel Button is disabled
Type | Default |
---|---|
object (style) | { color: '#cdcdcd' } |
cancelButtonTitle
(iOS only) title of the cancel button on the right side
Type | Default |
---|---|
string | "Cancel" |
cancelIcon
(platform="android"
only)
This props allows to override the Icon
props or use a custom component. Use
null
or false
to hide the icon.
Type | Default |
---|---|
{...Icon props} OR component | none |
clearIcon
This props allows to override the Icon
props or use a custom component. Use
null
or false
to hide the icon.
Type | Default |
---|---|
{...Icon props} OR component | none |
containerStyle
style the container of the SearchBar
Type | Default |
---|---|
object (style) | inherited styling |
inputContainerStyle
style the container of the TextInput
Type | Default |
---|---|
object (style) | inherited styling |
inputStyle
style the TextInput
Type | Default |
---|---|
object (style) | inherited styling |
leftIconContainerStyle
style the icon container on the left side
Type | Default |
---|---|
object (style) | inherited styling |
lightTheme
(platform="default"
only)
change theme to light theme
Type | Default |
---|---|
boolean | false |
loadingProps
props passed to ActivityIndicator
Type | Default |
---|---|
object | { } |
onCancel
callback fired when pressing the cancel button (iOS) or the back icon (Android)
Type | Default |
---|---|
function | null |
onChangeText
method to fire when text is changed
Type | Default |
---|---|
function | none |
onClear
method to fire when input is cleared
Type | Default |
---|---|
function | none |
placeholder
set the placeholder text
Type | Default |
---|---|
string | '' |
placeholderTextColor
set the color of the placeholder text
Type | Default |
---|---|
string | '#86939e' |
platform
choose the look and feel of the search bar. One of "default", "ios", "android"
Type | Default |
---|---|
string | "default" |
rightIconContainerStyle
style the icon container on the right side
Type | Default |
---|---|
object (style) | inherited styling |
round
(platform="default"
only)
change TextInput styling to rounded corners
Type | Default |
---|---|
boolean | false |
searchIcon
This props allows to override the Icon
props or use a custom component. Use
null
or false
to hide the icon.
Type | Default |
---|---|
{...Icon props} OR component | none |
showCancel
(platform="ios"
only)
When true
the cancel button will stay visible after blur events.
Type | Default |
---|---|
boolean | false |
showLoading
show the loading ActivityIndicator effect
Type | Default |
---|---|
boolean | false |
underlineColorAndroid
specify other than the default transparent underline color
Type | Default |
---|---|
string (color) | transparent |
value
The value of the search field
Type | Default |
---|---|
string | none |